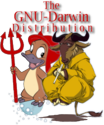
|
Main Page
Widgets
Namespaces
Book
Enums and Flags
|
Enumerations |
enum | CursorType {
X_CURSOR = 0,
ARROW = 2,
BASED_ARROW_DOWN = 4,
BASED_ARROW_UP = 6,
BOAT = 8,
BOGOSITY = 10,
BOTTOM_LEFT_CORNER = 12,
BOTTOM_RIGHT_CORNER = 14,
BOTTOM_SIDE = 16,
BOTTOM_TEE = 18,
BOX_SPIRAL = 20,
CENTER_PTR = 22,
CIRCLE = 24,
CLOCK = 26,
COFFEE_MUG = 28,
CROSS = 30,
CROSS_REVERSE = 32,
CROSSHAIR = 34,
DIAMOND_CROSS = 36,
DOT = 38,
DOTBOX = 40,
DOUBLE_ARROW = 42,
DRAFT_LARGE = 44,
DRAFT_SMALL = 46,
DRAPED_BOX = 48,
EXCHANGE = 50,
FLEUR = 52,
GOBBLER = 54,
GUMBY = 56,
HAND1 = 58,
HAND2 = 60,
HEART = 62,
ICON = 64,
IRON_CROSS = 66,
LEFT_PTR = 68,
LEFT_SIDE = 70,
LEFT_TEE = 72,
LEFTBUTTON = 74,
LL_ANGLE = 76,
LR_ANGLE = 78,
MAN = 80,
MIDDLEBUTTON = 82,
MOUSE = 84,
PENCIL = 86,
PIRATE = 88,
PLUS = 90,
QUESTION_ARROW = 92,
RIGHT_PTR = 94,
RIGHT_SIDE = 96,
RIGHT_TEE = 98,
RIGHTBUTTON = 100,
RTL_LOGO = 102,
SAILBOAT = 104,
SB_DOWN_ARROW = 106,
SB_H_DOUBLE_ARROW = 108,
SB_LEFT_ARROW = 110,
SB_RIGHT_ARROW = 112,
SB_UP_ARROW = 114,
SB_V_DOUBLE_ARROW = 116,
SHUTTLE = 118,
SIZING = 120,
SPIDER = 122,
SPRAYCAN = 124,
STAR = 126,
TARGET = 128,
TCROSS = 130,
TOP_LEFT_ARROW = 132,
TOP_LEFT_CORNER = 134,
TOP_RIGHT_CORNER = 136,
TOP_SIDE = 138,
TOP_TEE = 140,
TREK = 142,
UL_ANGLE = 144,
UMBRELLA = 146,
UR_ANGLE = 148,
WATCH = 150,
XTERM = 152,
LAST_CURSOR = 153,
CURSOR_IS_PIXMAP = -1
} |
enum | InputMode {
MODE_DISABLED,
MODE_SCREEN,
MODE_WINDOW
} |
enum | DragAction {
ACTION_DEFAULT = 1 << 0,
ACTION_COPY = 1 << 1,
ACTION_MOVE = 1 << 2,
ACTION_LINK = 1 << 3,
ACTION_PRIVATE = 1 << 4,
ACTION_ASK = 1 << 5
} |
enum | DragProtocol {
DRAG_PROTO_MOTIF,
DRAG_PROTO_XDND,
DRAG_PROTO_NONE,
DRAG_PROTO_WIN32_DROPFILES,
DRAG_PROTO_OLE2,
DRAG_PROTO_LOCAL
} |
enum | RgbDither {
RGB_DITHER_NONE,
RGB_DITHER_NORMAL,
RGB_DITHER_MAX
} |
enum | EventType {
NOTHING = -1,
DELETE,
DESTROY,
EXPOSE,
MOTION_NOTIFY,
BUTTON_PRESS,
DOUBLE_BUTTON_PRESS,
TRIPLE_BUTTON_PRESS,
BUTTON_RELEASE,
KEY_PRESS,
KEY_RELEASE,
ENTER_NOTIFY,
LEAVE_NOTIFY,
FOCUS_CHANGE,
CONFIGURE,
MAP,
UNMAP,
PROPERTY_NOTIFY,
SELECTION_CLEAR,
SELECTION_REQUEST,
SELECTION_NOTIFY,
PROXIMITY_IN,
PROXIMITY_OUT,
DRAG_ENTER,
DRAG_LEAVE,
DRAG_MOTION,
DRAG_STATUS,
DROP_START,
DROP_FINISHED,
CLIENT_EVENT,
VISIBILITY_NOTIFY,
NO_EXPOSE,
SCROLL,
WINDOW_STATE,
SETTING
} |
enum | ExtensionMode {
EXTENSION_EVENTS_NONE,
EXTENSION_EVENTS_ALL,
EXTENSION_EVENTS_CURSOR
} |
enum | AxisUse {
AXIS_IGNORE,
AXIS_X,
AXIS_Y,
AXIS_PRESSURE,
AXIS_XTILT,
AXIS_YTILT,
AXIS_WHEEL,
AXIS_LAST
} |
enum | LineStyle {
LINE_SOLID,
LINE_ON_OFF_DASH,
LINE_DOUBLE_DASH
} |
enum | CapStyle {
CAP_NOT_LAST,
CAP_BUTT,
CAP_ROUND,
CAP_PROJECTING
} |
enum | JoinStyle {
JOIN_MITER,
JOIN_ROUND,
JOIN_BEVEL
} |
enum | Fill {
SOLID,
TILED,
STIPPLED,
OPAQUE_STIPPLED
} |
enum | Function {
COPY,
INVERT,
XOR,
CLEAR,
AND,
AND_REVERSE,
AND_INVERT,
NOOP,
OR,
EQUIV,
OR_REVERSE,
COPY_INVERT,
OR_INVERT,
NAND,
NOR,
SET
} |
enum | SubwindowMode {
CLIP_BY_CHILDREN,
INCLUDE_INFERIORS
} |
enum | GCValuesMask {
GC_FOREGROUND = 1 << 0,
GC_BACKGROUND = 1 << 1,
GC_FONT = 1 << 2,
GC_FUNCTION = 1 << 3,
GC_FILL = 1 << 4,
GC_TILE = 1 << 5,
GC_STIPPLE = 1 << 6,
GC_CLIP_MASK = 1 << 7,
GC_SUBWINDOW = 1 << 8,
GC_TS_X_ORIGIN = 1 << 9,
GC_TS_Y_ORIGIN = 1 << 10,
GC_CLIP_X_ORIGIN = 1 << 11,
GC_CLIP_Y_ORIGIN = 1 << 12,
GC_EXPOSURES = 1 << 13,
GC_LINE_WIDTH = 1 << 14,
GC_LINE_STYLE = 1 << 15,
GC_CAP_STYLE = 1 << 16,
GC_JOIN_STYLE = 1 << 17
} |
enum | ImageType {
IMAGE_NORMAL,
IMAGE_SHARED,
IMAGE_FASTEST
} |
enum | Colorspace { COLORSPACE_RGB
} |
enum | InterpType {
INTERP_NEAREST,
INTERP_TILES,
INTERP_BILINEAR,
INTERP_HYPER
} |
enum | PixbufAlphaMode {
PIXBUF_ALPHA_BILEVEL,
PIXBUF_ALPHA_FULL
} |
enum | FillRule {
EVEN_ODD_RULE,
WINDING_RULE
} |
enum | OverlapType {
OVERLAP_RECTANGLE_IN,
OVERLAP_RECTANGLE_OUT,
OVERLAP_RECTANGLE_PART
} |
enum | ByteOrder {
LSB_FIRST,
MSB_FIRST
} |
enum | ModifierType {
SHIFT_MASK = 1 << 0,
LOCK_MASK = 1 << 1,
CONTROL_MASK = 1 << 2,
MOD1_MASK = 1 << 3,
MOD2_MASK = 1 << 4,
MOD3_MASK = 1 << 5,
MOD4_MASK = 1 << 6,
MOD5_MASK = 1 << 7,
BUTTON1_MASK = 1 << 8,
BUTTON2_MASK = 1 << 9,
BUTTON3_MASK = 1 << 10,
BUTTON4_MASK = 1 << 11,
BUTTON5_MASK = 1 << 12,
RELEASE_MASK = 1 << 30,
MODIFIER_MASK = 0x40001FFF
} |
enum | Status {
OK = 0,
ERROR = -1,
ERROR_PARAM = -2,
ERROR_FILE = -3,
ERROR_MEM = -4
} |
enum | InputCondition {
INPUT_READ = 1 << 0,
INPUT_WRITE = 1 << 1,
INPUT_EXCEPTION = 1 << 2
} |
enum | VisualType {
VISUAL_STATIC_GRAY,
VISUAL_GRAYSCALE,
VISUAL_STATIC_COLOR,
VISUAL_PSEUDO_COLOR,
VISUAL_TRUE_COLOR,
VISUAL_DIRECT_COLOR
} |
enum | EventMask {
EXPOSURE_MASK = 1 << 1,
POINTER_MOTION_MASK = 1 << 2,
POINTER_MOTION_HINT_MASK = 1 << 3,
BUTTON_MOTION_MASK = 1 << 4,
BUTTON1_MOTION_MASK = 1 << 5,
BUTTON2_MOTION_MASK = 1 << 6,
BUTTON3_MOTION_MASK = 1 << 7,
BUTTON_PRESS_MASK = 1 << 8,
BUTTON_RELEASE_MASK = 1 << 9,
KEY_PRESS_MASK = 1 << 10,
KEY_RELEASE_MASK = 1 << 11,
ENTER_NOTIFY_MASK = 1 << 12,
LEAVE_NOTIFY_MASK = 1 << 13,
FOCUS_CHANGE_MASK = 1 << 14,
STRUCTURE_MASK = 1 << 15,
PROPERTY_CHANGE_MASK = 1 << 16,
VISIBILITY_NOTIFY_MASK = 1 << 17,
PROXIMITY_IN_MASK = 1 << 18,
PROXIMITY_OUT_MASK = 1 << 19,
SUBSTRUCTURE_MASK = 1 << 20,
SCROLL_MASK = 1 << 21,
ALL_EVENTS_MASK = 0x3FFFFE
} |
enum | WindowState {
WINDOW_STATE_WITHDRAWN = 1 << 0,
WINDOW_STATE_ICONIFIED = 1 << 1,
WINDOW_STATE_MAXIMIZED = 1 << 2,
WINDOW_STATE_STICKY = 1 << 3,
WINDOW_STATE_FULLSCREEN = 1 << 4
} |
enum | WindowType {
WINDOW_ROOT,
WINDOW_TOPLEVEL,
WINDOW_CHILD,
WINDOW_DIALOG,
WINDOW_TEMP,
WINDOW_FOREIGN
} |
enum | WindowAttributesType {
WA_TITLE = 1 << 1,
WA_X = 1 << 2,
WA_Y = 1 << 3,
WA_CURSOR = 1 << 4,
WA_COLORMAP = 1 << 5,
WA_VISUAL = 1 << 6,
WA_WMCLASS = 1 << 7,
WA_NOREDIR = 1 << 8
} |
enum | WindowHints {
HINT_POS = 1 << 0,
HINT_MIN_SIZE = 1 << 1,
HINT_MAX_SIZE = 1 << 2,
HINT_BASE_SIZE = 1 << 3,
HINT_ASPECT = 1 << 4,
HINT_RESIZE_INC = 1 << 5,
HINT_WIN_GRAVITY = 1 << 6,
HINT_USER_POS = 1 << 7,
HINT_USER_SIZE = 1 << 8
} |
enum | WindowTypeHint {
WINDOW_TYPE_HINT_NORMAL,
WINDOW_TYPE_HINT_DIALOG,
WINDOW_TYPE_HINT_MENU,
WINDOW_TYPE_HINT_TOOLBAR,
WINDOW_TYPE_HINT_SPLASHSCREEN,
WINDOW_TYPE_HINT_UTILITY,
WINDOW_TYPE_HINT_DOCK,
WINDOW_TYPE_HINT_DESKTOP
} |
enum | WMDecoration {
DECOR_ALL = 1 << 0,
DECOR_BORDER = 1 << 1,
DECOR_RESIZEH = 1 << 2,
DECOR_TITLE = 1 << 3,
DECOR_MENU = 1 << 4,
DECOR_MINIMIZE = 1 << 5,
DECOR_MAXIMIZE = 1 << 6
} |
enum | WMFunction {
FUNC_ALL = 1 << 0,
FUNC_RESIZE = 1 << 1,
FUNC_MOVE = 1 << 2,
FUNC_MINIMIZE = 1 << 3,
FUNC_MAXIMIZE = 1 << 4,
FUNC_CLOSE = 1 << 5
} |
enum | WindowEdge {
WINDOW_EDGE_NORTH_WEST,
WINDOW_EDGE_NORTH,
WINDOW_EDGE_NORTH_EAST,
WINDOW_EDGE_WEST,
WINDOW_EDGE_EAST,
WINDOW_EDGE_SOUTH_WEST,
WINDOW_EDGE_SOUTH,
WINDOW_EDGE_SOUTH_EAST
} |
enum | Gravity {
GRAVITY_NORTH_WEST = 1,
GRAVITY_NORTH,
GRAVITY_NORTH_EAST,
GRAVITY_WEST,
GRAVITY_CENTER,
GRAVITY_EAST,
GRAVITY_SOUTH_WEST,
GRAVITY_SOUTH,
GRAVITY_SOUTH_EAST,
GRAVITY_STATIC
} |
enum | GrabStatus {
GRAB_SUCCESS,
GRAB_ALREADY_GRABBED,
GRAB_INVALID_TIME,
GRAB_NOT_VIEWABLE,
GRAB_FROZEN
} |
Functions |
DragAction | operator| (DragAction lhs, DragAction rhs) |
DragAction | operator& (DragAction lhs, DragAction rhs) |
DragAction | operator^ (DragAction lhs, DragAction rhs) |
DragAction | operator~ (DragAction flags) |
DragAction& | operator|= (DragAction& lhs, DragAction rhs) |
DragAction& | operator&= (DragAction& lhs, DragAction rhs) |
DragAction& | operator^= (DragAction& lhs, DragAction rhs) |
GCValuesMask | operator| (GCValuesMask lhs, GCValuesMask rhs) |
GCValuesMask | operator& (GCValuesMask lhs, GCValuesMask rhs) |
GCValuesMask | operator^ (GCValuesMask lhs, GCValuesMask rhs) |
GCValuesMask | operator~ (GCValuesMask flags) |
GCValuesMask& | operator|= (GCValuesMask& lhs, GCValuesMask rhs) |
GCValuesMask& | operator&= (GCValuesMask& lhs, GCValuesMask rhs) |
GCValuesMask& | operator^= (GCValuesMask& lhs, GCValuesMask rhs) |
ModifierType | operator| (ModifierType lhs, ModifierType rhs) |
ModifierType | operator& (ModifierType lhs, ModifierType rhs) |
ModifierType | operator^ (ModifierType lhs, ModifierType rhs) |
ModifierType | operator~ (ModifierType flags) |
ModifierType& | operator|= (ModifierType& lhs, ModifierType rhs) |
ModifierType& | operator&= (ModifierType& lhs, ModifierType rhs) |
ModifierType& | operator^= (ModifierType& lhs, ModifierType rhs) |
InputCondition | operator| (InputCondition lhs, InputCondition rhs) |
InputCondition | operator& (InputCondition lhs, InputCondition rhs) |
InputCondition | operator^ (InputCondition lhs, InputCondition rhs) |
InputCondition | operator~ (InputCondition flags) |
InputCondition& | operator|= (InputCondition& lhs, InputCondition rhs) |
InputCondition& | operator&= (InputCondition& lhs, InputCondition rhs) |
InputCondition& | operator^= (InputCondition& lhs, InputCondition rhs) |
EventMask | operator| (EventMask lhs, EventMask rhs) |
EventMask | operator& (EventMask lhs, EventMask rhs) |
EventMask | operator^ (EventMask lhs, EventMask rhs) |
EventMask | operator~ (EventMask flags) |
EventMask& | operator|= (EventMask& lhs, EventMask rhs) |
EventMask& | operator&= (EventMask& lhs, EventMask rhs) |
EventMask& | operator^= (EventMask& lhs, EventMask rhs) |
WindowState | operator| (WindowState lhs, WindowState rhs) |
WindowState | operator& (WindowState lhs, WindowState rhs) |
WindowState | operator^ (WindowState lhs, WindowState rhs) |
WindowState | operator~ (WindowState flags) |
WindowState& | operator|= (WindowState& lhs, WindowState rhs) |
WindowState& | operator&= (WindowState& lhs, WindowState rhs) |
WindowState& | operator^= (WindowState& lhs, WindowState rhs) |
WindowAttributesType | operator| (WindowAttributesType lhs, WindowAttributesType rhs) |
WindowAttributesType | operator& (WindowAttributesType lhs, WindowAttributesType rhs) |
WindowAttributesType | operator^ (WindowAttributesType lhs, WindowAttributesType rhs) |
WindowAttributesType | operator~ (WindowAttributesType flags) |
WindowAttributesType& | operator|= (WindowAttributesType& lhs, WindowAttributesType rhs) |
WindowAttributesType& | operator&= (WindowAttributesType& lhs, WindowAttributesType rhs) |
WindowAttributesType& | operator^= (WindowAttributesType& lhs, WindowAttributesType rhs) |
WindowHints | operator| (WindowHints lhs, WindowHints rhs) |
WindowHints | operator& (WindowHints lhs, WindowHints rhs) |
WindowHints | operator^ (WindowHints lhs, WindowHints rhs) |
WindowHints | operator~ (WindowHints flags) |
WindowHints& | operator|= (WindowHints& lhs, WindowHints rhs) |
WindowHints& | operator&= (WindowHints& lhs, WindowHints rhs) |
WindowHints& | operator^= (WindowHints& lhs, WindowHints rhs) |
WMDecoration | operator| (WMDecoration lhs, WMDecoration rhs) |
WMDecoration | operator& (WMDecoration lhs, WMDecoration rhs) |
WMDecoration | operator^ (WMDecoration lhs, WMDecoration rhs) |
WMDecoration | operator~ (WMDecoration flags) |
WMDecoration& | operator|= (WMDecoration& lhs, WMDecoration rhs) |
WMDecoration& | operator&= (WMDecoration& lhs, WMDecoration rhs) |
WMDecoration& | operator^= (WMDecoration& lhs, WMDecoration rhs) |
WMFunction | operator| (WMFunction lhs, WMFunction rhs) |
WMFunction | operator& (WMFunction lhs, WMFunction rhs) |
WMFunction | operator^ (WMFunction lhs, WMFunction rhs) |
WMFunction | operator~ (WMFunction flags) |
WMFunction& | operator|= (WMFunction& lhs, WMFunction rhs) |
WMFunction& | operator&= (WMFunction& lhs, WMFunction rhs) |
WMFunction& | operator^= (WMFunction& lhs, WMFunction rhs) |
Enumeration Type Documentation
|
- Enumeration values:
-
AXIS_IGNORE |
|
AXIS_X |
|
AXIS_Y |
|
AXIS_PRESSURE |
|
AXIS_XTILT |
|
AXIS_YTILT |
|
AXIS_WHEEL |
|
AXIS_LAST |
|
|
|
- Enumeration values:
-
CAP_NOT_LAST |
|
CAP_BUTT |
|
CAP_ROUND |
|
CAP_PROJECTING |
|
|
|
- Enumeration values:
-
X_CURSOR |
|
ARROW |
|
BASED_ARROW_DOWN |
|
BASED_ARROW_UP |
|
BOAT |
|
BOGOSITY |
|
BOTTOM_LEFT_CORNER |
|
BOTTOM_RIGHT_CORNER |
|
BOTTOM_SIDE |
|
BOTTOM_TEE |
|
BOX_SPIRAL |
|
CENTER_PTR |
|
CIRCLE |
|
CLOCK |
|
COFFEE_MUG |
|
CROSS |
|
CROSS_REVERSE |
|
CROSSHAIR |
|
DIAMOND_CROSS |
|
DOT |
|
DOTBOX |
|
DOUBLE_ARROW |
|
DRAFT_LARGE |
|
DRAFT_SMALL |
|
DRAPED_BOX |
|
EXCHANGE |
|
FLEUR |
|
GOBBLER |
|
GUMBY |
|
HAND1 |
|
HAND2 |
|
HEART |
|
ICON |
|
IRON_CROSS |
|
LEFT_PTR |
|
LEFT_SIDE |
|
LEFT_TEE |
|
LEFTBUTTON |
|
LL_ANGLE |
|
LR_ANGLE |
|
MAN |
|
MIDDLEBUTTON |
|
MOUSE |
|
PENCIL |
|
PIRATE |
|
PLUS |
|
QUESTION_ARROW |
|
RIGHT_PTR |
|
RIGHT_SIDE |
|
RIGHT_TEE |
|
RIGHTBUTTON |
|
RTL_LOGO |
|
SAILBOAT |
|
SB_DOWN_ARROW |
|
SB_H_DOUBLE_ARROW |
|
SB_LEFT_ARROW |
|
SB_RIGHT_ARROW |
|
SB_UP_ARROW |
|
SB_V_DOUBLE_ARROW |
|
SHUTTLE |
|
SIZING |
|
SPIDER |
|
SPRAYCAN |
|
STAR |
|
TARGET |
|
TCROSS |
|
TOP_LEFT_ARROW |
|
TOP_LEFT_CORNER |
|
TOP_RIGHT_CORNER |
|
TOP_SIDE |
|
TOP_TEE |
|
TREK |
|
UL_ANGLE |
|
UMBRELLA |
|
UR_ANGLE |
|
WATCH |
|
XTERM |
|
LAST_CURSOR |
|
CURSOR_IS_PIXMAP |
|
|
|
- Enumeration values:
-
DRAG_PROTO_MOTIF |
|
DRAG_PROTO_XDND |
|
DRAG_PROTO_NONE |
|
DRAG_PROTO_WIN32_DROPFILES |
|
DRAG_PROTO_OLE2 |
|
DRAG_PROTO_LOCAL |
|
|
|
- Enumeration values:
-
NOTHING |
|
DELETE |
|
DESTROY |
|
EXPOSE |
|
MOTION_NOTIFY |
|
BUTTON_PRESS |
|
DOUBLE_BUTTON_PRESS |
|
TRIPLE_BUTTON_PRESS |
|
BUTTON_RELEASE |
|
KEY_PRESS |
|
KEY_RELEASE |
|
ENTER_NOTIFY |
|
LEAVE_NOTIFY |
|
FOCUS_CHANGE |
|
CONFIGURE |
|
MAP |
|
UNMAP |
|
PROPERTY_NOTIFY |
|
SELECTION_CLEAR |
|
SELECTION_REQUEST |
|
SELECTION_NOTIFY |
|
PROXIMITY_IN |
|
PROXIMITY_OUT |
|
DRAG_ENTER |
|
DRAG_LEAVE |
|
DRAG_MOTION |
|
DRAG_STATUS |
|
DROP_START |
|
DROP_FINISHED |
|
CLIENT_EVENT |
|
VISIBILITY_NOTIFY |
|
NO_EXPOSE |
|
SCROLL |
|
WINDOW_STATE |
|
SETTING |
|
|
|
- Enumeration values:
-
EXTENSION_EVENTS_NONE |
|
EXTENSION_EVENTS_ALL |
|
EXTENSION_EVENTS_CURSOR |
|
|
|
- Enumeration values:
-
SOLID |
|
TILED |
|
STIPPLED |
|
OPAQUE_STIPPLED |
|
|
|
- Enumeration values:
-
EVEN_ODD_RULE |
|
WINDING_RULE |
|
|
|
- Enumeration values:
-
COPY |
|
INVERT |
|
XOR |
|
CLEAR |
|
AND |
|
AND_REVERSE |
|
AND_INVERT |
|
NOOP |
|
OR |
|
EQUIV |
|
OR_REVERSE |
|
COPY_INVERT |
|
OR_INVERT |
|
NAND |
|
NOR |
|
SET |
|
|
|
- Enumeration values:
-
GRAB_SUCCESS |
|
GRAB_ALREADY_GRABBED |
|
GRAB_INVALID_TIME |
|
GRAB_NOT_VIEWABLE |
|
GRAB_FROZEN |
|
|
|
- Enumeration values:
-
GRAVITY_NORTH_WEST |
|
GRAVITY_NORTH |
|
GRAVITY_NORTH_EAST |
|
GRAVITY_WEST |
|
GRAVITY_CENTER |
|
GRAVITY_EAST |
|
GRAVITY_SOUTH_WEST |
|
GRAVITY_SOUTH |
|
GRAVITY_SOUTH_EAST |
|
GRAVITY_STATIC |
|
|
|
- Enumeration values:
-
IMAGE_NORMAL |
|
IMAGE_SHARED |
|
IMAGE_FASTEST |
|
|
|
- Enumeration values:
-
MODE_DISABLED |
|
MODE_SCREEN |
|
MODE_WINDOW |
|
|
|
- Enumeration values:
-
INTERP_NEAREST |
|
INTERP_TILES |
|
INTERP_BILINEAR |
|
INTERP_HYPER |
|
|
|
- Enumeration values:
-
JOIN_MITER |
|
JOIN_ROUND |
|
JOIN_BEVEL |
|
|
|
- Enumeration values:
-
LINE_SOLID |
|
LINE_ON_OFF_DASH |
|
LINE_DOUBLE_DASH |
|
|
|
- Enumeration values:
-
OVERLAP_RECTANGLE_IN |
|
OVERLAP_RECTANGLE_OUT |
|
OVERLAP_RECTANGLE_PART |
|
|
enum Gdk::PixbufAlphaMode
|
|
|
- Enumeration values:
-
PIXBUF_ALPHA_BILEVEL |
|
PIXBUF_ALPHA_FULL |
|
|
|
- Enumeration values:
-
RGB_DITHER_NONE |
|
RGB_DITHER_NORMAL |
|
RGB_DITHER_MAX |
|
|
|
- Enumeration values:
-
OK |
|
ERROR |
|
ERROR_PARAM |
|
ERROR_FILE |
|
ERROR_MEM |
|
|
|
- Enumeration values:
-
CLIP_BY_CHILDREN |
|
INCLUDE_INFERIORS |
|
|
|
- Enumeration values:
-
VISUAL_STATIC_GRAY |
|
VISUAL_GRAYSCALE |
|
VISUAL_STATIC_COLOR |
|
VISUAL_PSEUDO_COLOR |
|
VISUAL_TRUE_COLOR |
|
VISUAL_DIRECT_COLOR |
|
|
enum Gdk::WindowAttributesType
|
|
|
- Enumeration values:
-
WINDOW_EDGE_NORTH_WEST |
|
WINDOW_EDGE_NORTH |
|
WINDOW_EDGE_NORTH_EAST |
|
WINDOW_EDGE_WEST |
|
WINDOW_EDGE_EAST |
|
WINDOW_EDGE_SOUTH_WEST |
|
WINDOW_EDGE_SOUTH |
|
WINDOW_EDGE_SOUTH_EAST |
|
|
|
- Enumeration values:
-
WINDOW_ROOT |
|
WINDOW_TOPLEVEL |
|
WINDOW_CHILD |
|
WINDOW_DIALOG |
|
WINDOW_TEMP |
|
WINDOW_FOREIGN |
|
|
|
- Enumeration values:
-
WINDOW_TYPE_HINT_NORMAL |
|
WINDOW_TYPE_HINT_DIALOG |
|
WINDOW_TYPE_HINT_MENU |
|
WINDOW_TYPE_HINT_TOOLBAR |
|
WINDOW_TYPE_HINT_SPLASHSCREEN |
|
WINDOW_TYPE_HINT_UTILITY |
|
WINDOW_TYPE_HINT_DOCK |
|
WINDOW_TYPE_HINT_DESKTOP |
|
|
Function Documentation
Generated for gtkmm by
Doxygen 1.3-rc1 © 1997-2001
|